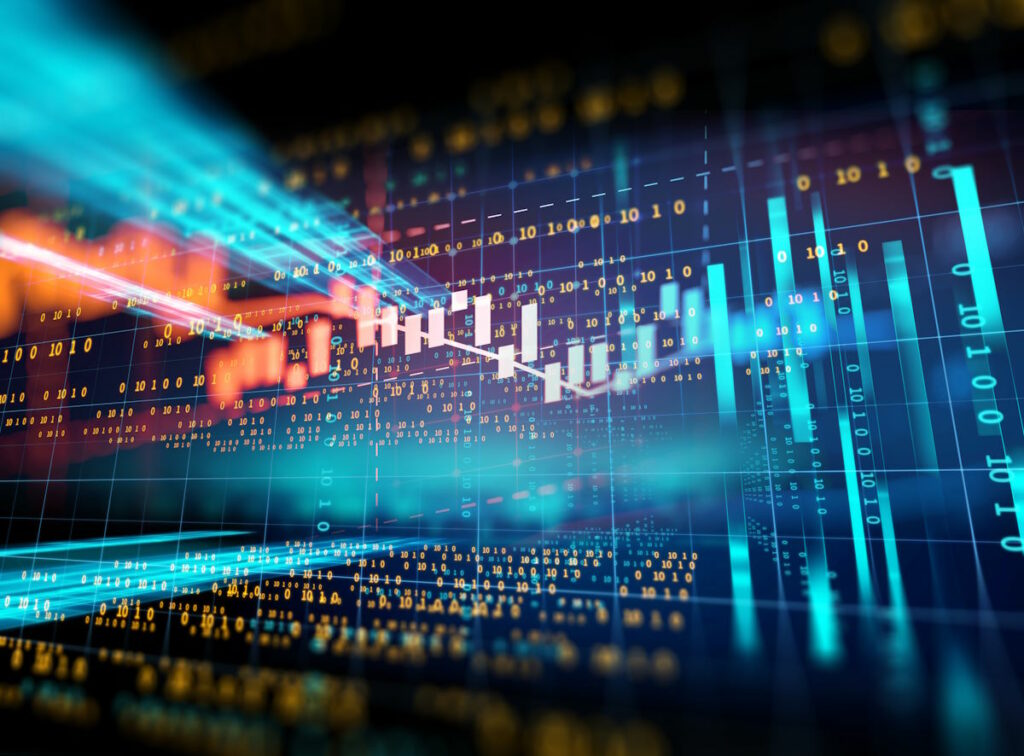
Introduction
The Moving Linear Regression Indicator, operates by creating an invisible line that best fits a specific set of data points. These points are determined by the Price and Period parameters and are calculated using a method known as the least squares technique. This method is designed to minimize the sum of the squares of the distances between each data point and the line.
The end point of this line, corresponding to the last point for the specified period, is then plotted on the chart. As we move to the next most recent bar, a new line is fitted to these points and the process repeats. The resulting Moving Linear Regression line is a connected series of these calculated end points.
What sets this indicator apart from other momentum-based, trend-following indicators is its ability to accurately track the trend, no matter its steepness or flatness. It maintains a high level of sensitivity without introducing time lag. Unlike other indicators, the MLR signals the end of a trend when the line changes direction, not when the price crosses the line.
The Moving Linear Regression Indicator is a tool that can be applied across different markets and timeframes. Typically, the variables are set between 20 and 80 periods, with the higher number being used for high frequency, short term day trading methods.
Additionally, bands can be placed around the line for further analysis. The preferred setting for this is the factor and standard error with a factor set at 2 or 3. This setting provides a fixed reference on an extreme, allowing for precise trend following trades or aggressive counter trend trades.
The Genesis of the Moving Linear Regression Indicator
While the exact date of its inception and the identity of its inventor remain elusive, the technique it employs, the least squares method, has been a cornerstone in the field of statistics for centuries.
The least squares method, which forms the basis of the MLR indicator, was first published by Carl Friedrich Gauss in 1809. This method is designed to find the best-fitting line to a set of data points by minimizing the sum of the squares of the distances from each point to the line.
The application of this statistical method to financial markets has provided traders with a powerful tool for tracking market trends. This indicator’s unique ability to maintain sensitivity to market changes, regardless of the steepness or flatness of the trend, sets it apart from other indicators.
The Mathematical Framework
The MLR Indicator is rooted in a mathematical technique known as linear regression. This technique is employed to calculate the best-fitting straight line through a set of data points. This line is computed in a manner that minimizes the sum of the squares of the distances (referred to as residuals) from each data point to the line itself.
The formula provided below is a simplified representation of the linear regression model, focusing on the calculation of the slope:
Slope (m) = Σ [ (Price – Mean(Price)) * (Period – Mean(Period)) ] / Σ [ (Period – Mean(Period))^2 ]
Where:
- Price refers to the price data points.
- Period refers to the number of periods.
- Mean(Price) is the average price over the specified period.
- Mean(Period) is the average value of the period numbers.
This formula computes the covariance of the price and the period (the numerator) and divides it by the variance of the period (the denominator). The result, the slope (m), gives us a measure of the rate of change in the dependent variable (Price) for each unit change in the independent variable (Period).
However, in practice, the indicator doesn’t plot this slope directly. Instead, for each time point, it uses the linear regression model fitted to the previous window of data (which includes both the slope and the intercept) to predict the value of the dependent variable (Price) for the next period. This predicted value is what we plot in the Moving Linear Regression Indicator. This methodology allows the MLR to more accurately capture the trend in the data, especially when the trend is not merely moving up or down at a constant rate.
In the upcoming Python code implementation, we will be using the sklearn library’s LinearRegression function to compute the coefficients for our linear regression model, including both the slope and the intercept, and then use these coefficients to predict the price for the next period.
Deciphering Moving Linear Regression Indicator Signals
The MLR Indicator provides valuable insights into market trends, but understanding how to interpret its output is crucial for making informed trading decisions. The indicator plots a line that best fits the data points over a specified period, and this line is used to track the trend of the market.
When the Moving Linear Regression line is moving upwards, it indicates an upward trend in the market, suggesting a potential buy signal. Conversely, when the line is moving downwards, it indicates a downward trend, suggesting a potential sell signal.
One unique feature of this indicator is that it signals the end of a trend when the line changes direction, not when the price crosses the line. Therefore, a change in the direction of the Moving Linear Regression line can be seen as a signal of a potential reversal in the market trend.
In real-world trading, it is often used in conjunction with other indicators to confirm signals and improve the accuracy of predictions. For example, traders may use the MLR indicator to track the overall trend of the market, and then use other indicators to identify the best entry and exit points for their trades.
The Strengths and Weaknesses
The Moving Linear Regression Indicator offers several benefits to traders. Its ability to track market trends regardless of their steepness or flatness allows it to maintain sensitivity to market changes without introducing time lag. This makes it a valuable tool for both long-term and short-term trading strategies.
While it is effective in trending markets, it may produce false signals in range-bound or sideways markets.
MLR vs Moving Averages
Moving averages and the Moving Linear Regression (MLR) are both popular tools in technical analysis, but they are calculated in different ways and provide different types of information.
Moving Averages: A moving average is calculated by adding up a certain number of past data points (usually closing prices) and then dividing by the number of data points. The result is a single line that smooths out price data by continuously creating an updated average price. The two most common types of moving averages are the Simple Moving Average (SMA) and the Exponential Moving Average (EMA). The SMA gives equal weight to all data points in the period, while the EMA gives more weight to recent data points.
Moving Linear Regression (MLR): The MLR, on the other hand, fits a straight line through a set of data points using the least squares method. The least squares method calculates the best-fitting line by minimizing the sum of the squares of the vertical distances of each data point from the line (these distances are the “errors”). The MLR line is a series of end points of these best-fitting lines, creating a line that can better fit trends that are not simply moving up or down at a constant rate.
In summary, while moving averages provide a smoothed average of price data, the Moving Linear Regression provides a line that best fits the data points, potentially offering a more accurate representation of trends that are not linear.
Implementing the MLR Indicator in Python with VSCode
Implementing the Moving Linear Regression Indicator in Python involves several steps. Here’s how you would do it with VSCode (free software from Microsoft). Install it and set up Python by following the step by step guide within it, then use the terminal window at the base of the screen to install the required libraries we will use:
Import Libraries:
pip install pandas yfinance mplfinance matplotlib numpy scikit-learn
Next save a new .py python file e.g. MLR.py and start placing the following code snippets into it:
import pandas as pd
import yfinance as yf
import mplfinance as mpf
import matplotlib.pyplot as plt
import matplotlib.lines as mlines
import numpy as np
from sklearn.linear_model import LinearRegression
Download Data:
Use the yfinance
library to download data from Yahoo Finance using the yf.download()
function. For example, to download data for the S&P 500:
data = yf.download('^GSPC', start='2022-07-30', end='2023-07-30')
Calculate Moving Linear Regression:
Next, calculate the Moving Linear Regression. For simplicity, we’ll use a 20-day moving window. Note that the Python implementation uses the sklearn’s LinearRegression function, which computes the coefficients for a linear regression model (including the slope and intercept), and then uses these coefficients to predict the price for the next time period.
def calculate_mlr(data, window_size):
mlr = [np.nan] * window_size
for i in range(window_size, len(data)):
y = data.iloc[i - window_size:i].values.reshape(-1, 1)
x = np.array(range(i - window_size, i)).reshape(-1, 1)
reg = LinearRegression().fit(x, y)
mlr.append(reg.predict(np.array([i]).reshape(-1, 1))[0][0])
return mlr
# Calculate MLR
data['MLR'] = calculate_mlr(data['Close'], 20)
After defining a function to calculate MLR, we apply it to our data. The ‘calculate_mlr’ function takes as input the data and a window size. For each point in time from the start of the window, it fits a linear regression model to the previous window of data. It then uses this model to predict the value of the dependent variable (Price) for the next period. This predicted value is appended to the ‘mlr’ list, which is then added to the original data as a new column. This process is done using the sklearn library’s LinearRegression function, which computes the coefficients for our linear regression model (including the slope and the intercept), and then uses these coefficients to predict the price for the next period.
Plot Data:
The next step is to create a plot for the data. You will be using mplfinance
to create a candlestick chart, and matplotlib
to add the Moving Linear Regression line to the chart:
- An addplot is created using
mpf.make_addplot()
with the MLR data fromdata['MLR']
and a specified color. - The candlestick chart is plotted using
mpf.plot()
, with the volume data enabled (volume=True
), the addplot (addplot=ap
), and the ‘yahoo’ style. The figure size is set to (10, 8), and a title is added.
# Create an addplot for the MLR
ap = mpf.make_addplot(data['MLR'], color='blue')
# Plot the candlestick chart with the volume and the MLR
fig, axes = mpf.plot(data, type='candle', volume=True, addplot=ap, style='yahoo', figratio=(10,8), title='S&P 500 - Moving Linear Regression', returnfig=True)
Create a Dummy Line for the Legend:
Next, create a dummy line to add to the legend. This line will represent the Moving Linear Regression line in the legend:
# Create a dummy line to add to the legend
mlr_line = mlines.Line2D([], [], color='blue', label='MLR')
This line of code creates a line with no points (hence the empty square brackets), a blue color, and a label of ‘MLR’.
Add the Legend to the Figure:
Finally, add the legend to the figure and adjust its position:
# Add the legend to the figure and adjust its position
leg = fig.legend(handles=[mlr_line], loc='center left', bbox_to_anchor=(0.05, 0.5))
This line of code adds a legend to the left of the figure, centered vertically. The bbox_to_anchor
argument specifies the position of the legend: (0.05, 0.5) means that the legend will be 5% from the left and 50% from the bottom of the figure.
Show the Plot:
The last step is to display the plot:
plt.show()
This line of code displays the plot in a new window.
If all has gone to plan you should end up with a chart generated that looks like mine shown below:
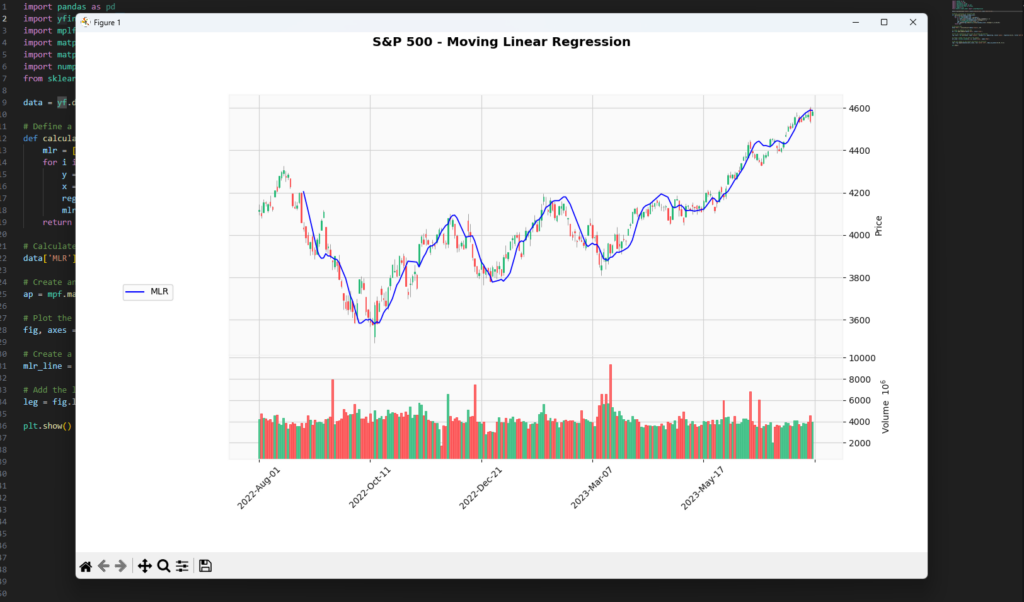
The blue line in the plot, which represents the Moving Linear Regression (MLR), is a line of predicted prices for the next time point based on the linear regression model fitted to the past data (in this case, the past 20 days).
Note that these predictions are based on historical data and do not account for real-time fluctuations in price that might occur during the trading period. The predicted price for the current candle is determined as soon as the candle opens, based on the available historical data up to the close of the previous candle. Once the new candle begins, the MLR value for that specific candle remains fixed and does not change, even if the actual price fluctuates within the duration of the candle. The MLR line will update only with the arrival of the next candle’s data, providing a new predicted value for the opening price of the next candle based on the updated historical data from the previous candles.
Practical Guidelines for Applying the Moving Linear Regression Indicator in Trading
- Understand the Indicator: Before using the indicator, make sure you understand what it measures and how it works. Familiarize yourself with the least squares technique and how the indicator uses this technique to track market trends.
- Use in Conjunction with Other Indicators: The indicator is most effective when used in conjunction with other indicators. For example, you might use the Moving Linear Regression Indicator to track the overall trend of the market, and then use other indicators to identify the best entry and exit points for your trades.
- Customize the Indicator to Your Needs: The indicator is flexible and can be customized to meet your specific needs. Experiment with different settings for the Price and Period parameters to find the combination that works best for your trading strategy.
- Be Aware of Its Unique Characteristics: It’s important to understand that the MLR line is not a conventional “lagging” indicator. While it is calculated based on past data, it is not a smoothed average like many other lagging indicators (e.g., moving averages). Instead, the MLR provides the predicted value for the current (or next) time period based on a linear regression model fitted to past data. This makes it potentially more responsive to recent changes in the data, providing a timely indication of changing trends.
MLR for Day Trading
For high-frequency or day trading, you may want to adjust the Moving Linear Regression Indicator to reflect the shorter timeframes and faster pace of these trading strategies. Here are some suggestions:
- Adjust the Period Parameter: The period parameter determines the number of data points used to calculate the Moving Linear Regression line. For high-frequency or day trading, you might want to use a shorter period to reflect the faster pace of trading. For example, you might use a 5 or 10-period Moving Linear Regression instead of the more common 20 or 50-period.
- Use Intraday Data: Kind of obvious but high-frequency and day trading involve making many trades within a single day. Therefore, you might want to use intraday data (e.g., 1-minute or 5-minute bars) instead of daily data when calculating it.
- Combine with Other Short-Term Indicators: The Moving Linear Regression Indicator can provide valuable insights into market trends, but it’s most effective when used in conjunction with other indicators. For high-frequency or day trading, consider using other short-term indicators, such as the Relative Strength Index (RSI) or the Moving Average Convergence Divergence (MACD), to confirm signals and improve the accuracy of your predictions.
- Be Aware of Market Noise: High-frequency and day trading involve a higher level of market noise, which can lead to more false signals. Be aware of this when interpreting the signals from it and consider using additional filters or confirmation from other indicators to improve the accuracy of your signals.
Difference Between Moving Linear Regression Indicator (MLR) and End Point Moving Average (EPMA)
Occasionally I see these two indicators interchangeably referred to, ‘also known as’. While both the MLR and EPMA use a window of data points and calculate a line through those points, they are fundamentally different in their methodologies and what they plot.
The MLR uses standard linear regression over a given window of data points and plots the predicted value for the next time point, i.e., the value of the regression line at the most recent point in the window.
On the other hand, the EPMA calculates a weighted moving average and plots this average. However, unlike conventional weighted moving averages where more recent data points have more weight, the weights in EPMA are determined by the distance of each data point from the end point. The data point furthest from the end point receives the smallest weight, while the one at the end point receives the largest weight.
So while these two indicators might appear similar and are sometimes used in similar contexts, they are not the same and shouldn’t be referred to interchangeably.
Wrapping Up
The Moving Linear Regression Indicator is notable amongst the plethora of available indicators. By employing the least squares technique, it creates a moving linear regression line that accurately tracks market trends, offering crucial insights into their direction and intensity. Its unique feature of signaling the end of a trend when the line changes direction, rather than when the price crosses the line, distinguishes it from other indicators.
We’ve explored the mathematical framework of the MLR, understanding its purpose and design, and how to decipher its signals. We’ve also examined its strengths and weaknesses, including a comparison with other similar indicators such as moving averages.
Furthermore, we’ve discussed how to implement the moving linear regression formula in Python using VSCode, providing a step-by-step guide and detailed examples of Python code. We’ve also offered practical tips for using it in trading, including adjustments for high-frequency or day trading.
Remember, the effectiveness of the Moving Linear Regression Line, like all tools, is dependent on how well it is used. Therefore, it’s crucial to understand the indicator, use it in conjunction with other indicators, customize it to your needs, be aware of its limitations, and always practice good risk management.
We encourage you to continue learning and experimenting with the Moving Linear Regression Indicator. The more you understand and practice with it, the more effectively you can use it in your trading. Tell us how you get on with it.
Leave a Reply