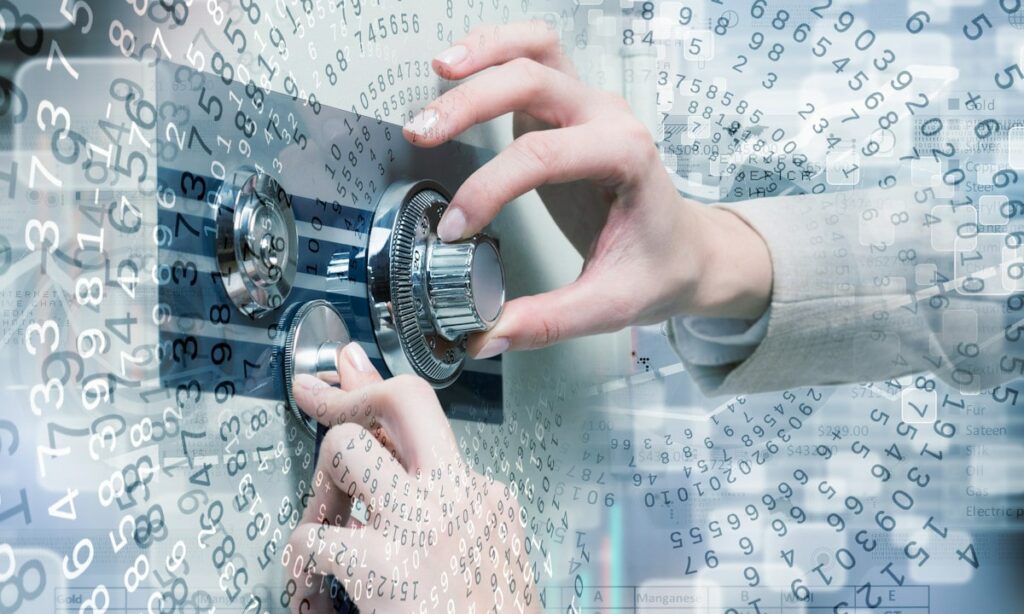
Origins of Klinger Volume Oscillator
The Klinger Volume Oscillator was developed by Stephen Klinger. The primary motivation behind its creation was to provide a comprehensive tool that could accurately measure the volume force in a market. The volume force is a key factor in market analysis as it can indicate the strength of a particular price movement. By developing the KVO, Klinger aimed to provide traders with a more nuanced understanding of market dynamics, thereby enabling them to make more informed trading decisions.
Mathematical Construction
The Klinger Volume Oscillator is designed by combining volume and price data to provide insights into market trends. The mathematical formula consists of several steps, including the calculation of key price, volume force, and the actual oscillator. Here’s how it’s calculated:
- Key Price (t): This is the average of the high, low, and closing prices for a given period (t). Key Price (t) = (High (t) + Low (t) + Close (t)) / 3
- Key Price (t-1): This is the average of the high, low, and closing prices for the previous period (t-1). Key Price (t-1) = (High (t-1) + Low (t-1) + Close (t-1)) / 3
- Volume Force (VF): This is calculated based on the comparison between the Key Price of the current period and the previous period. If the current Key Price is greater than the previous Key Price, the Volume Force is equal to the Volume; otherwise, it’s negative Volume. VF = Volume if Key Price_t > Key Price_t-1, otherwise -Volume
- KVO: The Klinger Volume Oscillator is calculated by subtracting the long-period Exponential Moving Average (EMA) of the Volume Force from the short-period EMA of the Volume Force. The short and long periods are typically 34 and 55, respectively. KVO = EMA_short-period (VF) – EMA_long-period (VF)
- KVO Signal Line: This is a moving average of the KVO, commonly calculated as a 13-period Exponential Moving Average (EMA) of the KVO. KVO Signal Line = EMA(13) (KVO)
- Klinger Oscillator Signal Line (KOS): This is the same as the KVO Signal Line. It’s used as a signal line for generating trading signals.
The Key Price helps in determining the direction of the volume force, while the KVO Signal Line and Klinger Oscillator Signal Line (KOS) provide a smoothed version of the KVO for generating trading signals. By analyzing these components, traders can gain insights into market trends, reversals, and potential trading opportunities.
Purpose and Design
The Klinger Volume Oscillator is designed to measure the volume force of a market. Volume force is a concept that combines price and volume to provide a more comprehensive view of market trends. By measuring volume force, the KVO can help traders identify potential reversals and confirm current trends.
The indicator is typically used in trading to identify divergence between price and volume trends. For example, if the price is increasing but the KVO is decreasing, it could indicate a potential price reversal. Conversely, if the price is decreasing and the KVO is increasing, it could suggest a bullish reversal.
One of the unique features of this indicator is the inclusion of a signal line, known as the Klinger Oscillator Signal line (KOS). This line is a 13-period EMA of the KVO and can be used to generate trading signals. When the KVO crosses above the KOS, it can be seen as a bullish signal, and when it crosses below the KOS, it can be seen as a bearish signal.
- Bullish Signals: A bullish signal is generated when the KVO line crosses above the Klinger Oscillator Signal line (KOS). This suggests that the volume force is increasing, indicating potential upward price movement. Traders might consider this an opportunity to open a long position.
- Bearish Signals: Conversely, a bearish signal is generated when the KVO line crosses below the KOS. This suggests that the volume force is decreasing, indicating potential downward price movement. In this case, traders might consider it an opportunity to open a short position.
- Divergence: Divergence between the KVO and price can also provide valuable trading signals. If the price is rising but the KVO is falling, it could indicate a bearish divergence and a potential upcoming price reversal. Similarly, if the price is falling but the KVO is rising, it could suggest a bullish divergence and a potential price increase.
Pros and Cons
Understanding the strengths and weaknesses of this indicator can help you effectively incorporate it into your trading strategy.
Advantages of Using Klinger Volume Oscillator
- Volume and Price Analysis: It offers a unique blend of volume and price data, providing a more holistic view of the market than indicators that consider price data alone.
- Spotting Divergences: It excels at identifying divergences between price and volume trends. These divergences can often signal potential reversals, offering early entry signals.
- Signal Line: The inclusion of the Klinger Oscillator Signal line (KOS) provides straightforward, easy-to-interpret trading signals.
Disadvantages or Limitations of Klinger Volume Oscillator
- Potential for False Signals: It can sometimes generate false signals. For instance, it might suggest a bullish divergence, but the price could continue to decline.
- Lagging Nature: It relies on past data, which means it can confirm trends and spot potential reversals, but it may not be as effective in predicting future price movements.
- Complexity: The mathematical formula behind it is quite intricate, which could be challenging for novice traders.
Coding Klinger Volume Oscillator into Trading Algorithms
Incorporating this into your trading algorithms can assist with providing valuable insights into market trends. Here’s a general guide on how you might do this:
- Define the Key Price: The Key Price is calculated as the average of the high, low, and closing prices for a given period (t) and the previous period (t-1).
- Calculate the Volume Force (VF): Determine the Volume Force based on the comparison of the Key Price of the current and previous periods. If the current Key Price is greater than the previous Key Price, the Volume Force is equal to the Volume; otherwise, it’s negative Volume.
- Calculate the KVO: The KVO is calculated by subtracting the long-period Exponential Moving Average (EMA) of the Volume Force from the short-period EMA of the Volume Force. The short and long periods are typically 34 and 55, respectively.
- Calculate the KVO Signal Line: The KVO Signal Line is a moving average of the KVO, commonly calculated as a 13-period Exponential Moving Average (EMA) of the KVO.
- Calculate the Klinger Oscillator Signal Line (KOS): The KOS is the same as the KVO Signal Line and is used for generating trading signals.
- Generate Trading Signals: Trading signals are generated based on the relationship between the KVO and the KOS. A bullish signal is generated when the KVO crosses above the KOS, and a bearish signal is generated when the KVO crosses below the KOS.
Remember, this is a simplified guide. The actual coding process will depend on the specific programming language you’re using (like Python or R) and the trading platform you’re working with. Always test your algorithm thoroughly before live trading.
Klinger Volume Oscillator in Python
So let’s use these principles to make the indicator in Python for a chosen stock and plot it on a chart, in this case I chose Apple stock. We are using VSCode from Microsoft which is free. Install python following the step by step instructions within VSCode and install the required libraries in the Terminal section using pip install.
1. Install the Required Libraries
If you haven’t already, install the necessary Python libraries using the following command:
pip install pandas yfinance mplfinance matplotlib numpy
Create a python file and (save it as klinger.py or similar) and start building the code below into it.
2. Import Necessary Libraries
Begin by importing the libraries needed. Pandas is used for data manipulation, yfinance for downloading stock data, mplfinance for creating financial charts, matplotlib for additional plotting functionality, and numpy for numerical operations.
import pandas as pd
import yfinance as yf
import mplfinance as mpf
import matplotlib.pyplot as plt
import matplotlib.lines as mlines
import numpy as np
3. Download Historical Data
The yfinance library is used to download historical data for a specific ticker symbol (in this case, ‘AAPL’ for Apple Inc.) for the time period from July 31, 2022, to July 31, 2023.
start_date = '2022-07-31'
end_date = '2023-07-31'
ticker = 'AAPL'
data = yf.download(ticker, start=start_date, end=end_date)
4. Define the Klinger Oscillator Function
We need to calculate the Key Price, Volume Force, KVO, and KVO Signal Line.
def klinger_oscillator(df, short_period=34, long_period=55, signal_period=13):
key_price_t = (df['High'] + df['Low'] + df['Close']) / 3
key_price_t_minus_1 = key_price_t.shift(1)
vf = np.where(key_price_t > key_price_t_minus_1, df['Volume'], -df['Volume'])
kvo = vf.ewm(span=short_period).mean() - vf.ewm(span=long_period).mean()
kvo_signal = kvo.ewm(span=signal_period).mean()
return kvo, kvo_signal
5. Calculate the KVO and Signal Line
Apply the Klinger Oscillator function to calculate the KVO and KVO Signal Line.
data['kvo'], data['kvo_signal'] = klinger_oscillator(data)
6. Clean the Data
Drop any rows with NaN values in the ‘kvo’ and ‘kvo_signal’ columns.
data = data.dropna(subset=['kvo', 'kvo_signal'])
7. Create Subplots for the KVO and Signal Line
The mplfinance library’s make_addplot
function is used to create subplots for the KVO and signal line. These subplots will be added to the main plot later.
kvo_plot = mpf.make_addplot(data['kvo'], panel=1, color='b')
kvo_signal_plot = mpf.make_addplot(data['kvo_signal'], panel=1, color='r')
8. Create the Candlestick Chart with it on
The mplfinance library’s plot
function is used to create a candlestick chart of the stock data, with the KVO and signal line subplots added below the main chart. The returnfig=True
argument is used to return the figure and axes objects, which are needed to add the legend in the next step.
fig, axes = mpf.plot(data, type='candle', style='yahoo', addplot=[kvo_plot, kvo_signal_plot], title='Apple Stock Price with Klinger Volume Oscillator', ylabel='Price ($)', ylabel_lower='KVO', volume=True, returnfig=True)
9. Add the Legend
Create dummy Line2D objects for the KVO and signal line, and add a legend to the last subplot.
kvo_line = mlines.Line2D([], [], color='b', label='KVO')
kvo_signal_line = mlines.Line2D([], [], color='r', label='KVO Signal')
axes[-1].legend(handles=[kvo_line, kvo_signal_line], loc='upper left')
10. Display the Plot
Finally, use plt.show() to display the plot.
plt.show()
If you’ve followed along you should hopefully have something that looks similar to mine when you press the play button in the top right after saving the file changes.
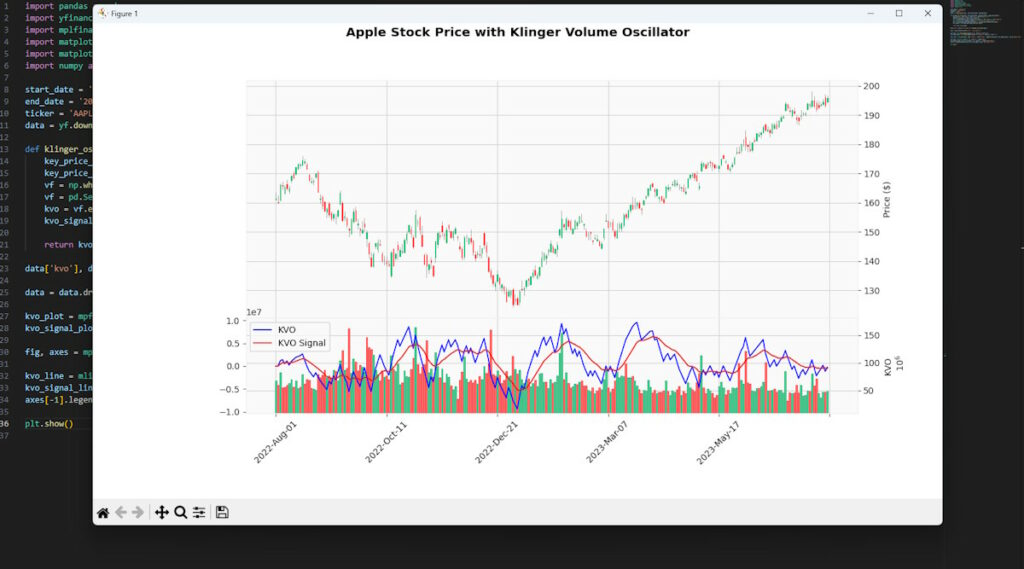
Not all KVO indicators on commercial trading platforms will necessarily look identical, even if we apply the same basic settings to them – typically 13, 34 and 55. Where the short cycle (short_period) is set to 34, the long cycle (long_period) is set to 55 and the signal line of the KVO as a 13-period moving average of the KVO. In our example we have followed to same construction as CQG IC uses.
For example although this oscillator is generally calculated in a standard way, different platforms might use slightly different methods or parameters. For example, they might handle missing data differently, or they might use a different type of moving average (e.g., simple vs. exponential) or they may add additional smoothing functions.
Frequently Asked Questions (FAQs)
- What is the Klinger Volume Oscillator (KVO)? It is is a technical indicator that combines volume and price data to provide a comprehensive view of market trends. It was developed by Stephen Klinger to help traders identify potential reversals and confirm current trends.
- How is the Klinger Volume Oscillator calculated? Using a complex formula that involves the calculation of a volume force, which is then used to calculate two exponential moving averages (EMAs). The KVO is the difference between these two EMAs.
- How do I use it in my trading strategy? It can be used to identify potential trading signals. A bullish signal is generated when the KVO line crosses above the Klinger Oscillator Signal line (KOS), and a bearish signal is generated when the KVO line crosses below the KOS. Divergences between the KVO and price can also provide valuable trading signals.
- What are the limitations of the Klinger Volume Oscillator? While it can provide valuable insights, it can sometimes generate false signals. It is also a lagging indicator, meaning it relies on past data and may not be as effective in predicting future price movements. The mathematical formula behind the KVO is quite intricate, which could be challenging for novice traders.
Key Takeaways
Navigating the world of trading indicators can be complex, but understanding the Klinger Volume Oscillator (KVO) can give you an edge. Here are the essential points to remember:
- Inventor’s Insight: Stephen Klinger’s creation, is a testament to the importance of volume force in market analysis. It’s a tool that goes beyond price action, offering a more nuanced understanding of market dynamics.
- Formula Behind the Scenes: The intricate calculation involves a unique concept of volume force and the use of two exponential moving averages (EMAs). This combination results in an oscillator that fluctuates around a zero line, providing valuable trading signals.
- Signal Interpretation: The KVO offers a way to interpret market trends. When the KVO line crosses the Klinger Oscillator Signal line (KOS), it’s time to pay attention. An upward cross can indicate a bullish trend, while a downward cross can signal a bearish trend. Spotting divergences between the KVO and price trends can also hint at potential reversals.
- Balancing Act: While the indicator brings the advantage of combining volume and price data and excels at spotting divergences, it’s important to be aware of its limitations. It can occasionally produce false signals, and its reliance on past data means it’s a lagging indicator. Also, its complex calculation might be a hurdle for beginners.
- Coding the KVO: Incorporating it into trading algorithms can streamline the process of spotting trading signals. The Python script provided in this guide is a practical example of how to achieve this using popular data analysis and visualization libraries.
Remember, this indicator is just one piece of the puzzle. A well-rounded trading strategy should consider multiple indicators and fundamental market analysis too.
Leave a Reply