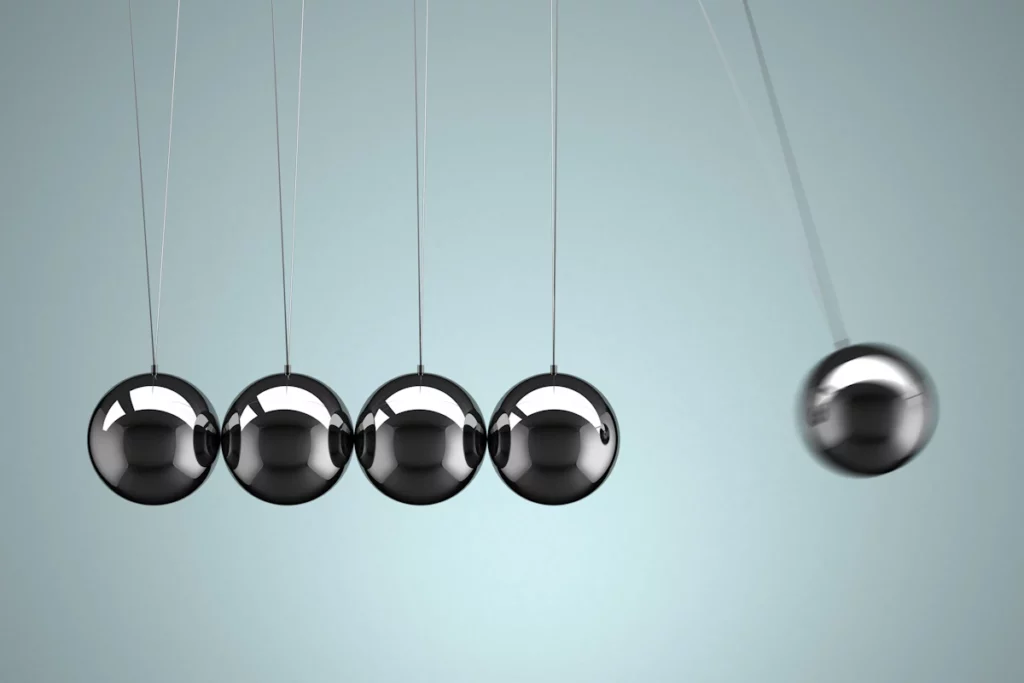
Introduction
The Intraday Momentum Index (IMI) is a technical indicator that combines elements of candlestick analysis with the relative strength index (RSI) to generate overbought or oversold signals. It is seen as a valuable indicator in technical analysis mainly due to its ability to provide insights into market momentum on an intraday basis. It is particularly useful for traders who engage in day trading or short-term trading strategies, as it can help identify potential entry and exit points based on market momentum over short timeframe horizons which assists with trade strategy styles such as scalping or jobbing.
Origins
The IMI was developed by the well known market technician, Tushar Chande in the 1990s. Chande developed the indicator to provide insights into intraday market momentum which he did by incorporating aspects of candlestick analysis with the RSI indicator to create it.
The indicator has since become more popular as technology advancements made intraday trading accessible to the masses and is now recognised as one of the best momentum indicators for day trading.
Mathematical Construction
The index calculation uses the following formula:
\mathrm{IMI}=\left(\frac{\sum_{p=1}^n \text { Gains }}{\sum_{p=1}^n \text { Gains }+\sum_{p=1}^n \text { Losses }}\right) \times 100
Where:
- Gains = CP − OP on Up Periods, i.e., Close > Open
- CP = Closing price
- OP = Opening price
- Losses= OP − CP on Down Periods, i.e., Open > Close
- p = periods (i.e. intraday candle sticks)
- n = Number of periods (14 is commonly used)
The IMI is calculated by first determining the gains and losses for each period over a certain number of periods (commonly 14 periods). If using intraday data such as 15-minute candles, the “period” would refer to each 15-minute interval, and “14 periods” would amount to 3.5 hours of trading data.
- A gain is calculated as the difference between the closing price and the opening price on candles when the closing price is higher than the opening price (Up Periods).
- A loss is calculated as the difference between the opening price and the closing price on candles when the opening price is higher than the closing price (Down Periods).
Once the gains and losses are calculated for each period, the total gains and total losses over the lookback period are calculated.
The IMI is then calculated as the total gains divided by the sum of the total gains and total losses, multiplied by 100 to express it as a percentage.
This percentage gives an indication of the momentum in the market, with higher values indicating stronger upward momentum and lower values indicating stronger downward momentum.
Interpreting Intraday Momentum Index Signals
Interpreting the IMI involves understanding what the calculated percentage means. A high IMI value (close to 100%) indicates strong upward momentum, suggesting that the market may be overbought. Meanwhile, a low IMI value (close to 0%) indicates strong downward momentum, suggesting that the market may be oversold.
Traders can use these signals to identify potential entry and exit points for their trades. For example, if the IMI is high and a trader expects the market to correct based on additional elements such as bearish candlestick formations, they might consider selling or shorting. On the other hand, if the IMI is low and the trader expects the market to rebound, they might consider buying.
Pros and Cons
Pros:
- Intraday Momentum Insights: The primary strength of the IMI lies in its ability to offer insights into intraday market momentum, assisting traders in gauging the strength of price movements within the trading day.
- Leading Indicator: Unlike some other technical indicators, the IMI is a leading indicator. This means it aims to predict future price movements rather than just reflecting past data.
- Versatility: The IMI can be used in conjunction with other technical indicators, pattern analysis, and order flow (especially beneficial for scalpers in futures trading) to refine trading strategies and enhance signal accuracy.
Cons:
- Potential False Signals: While the IMI provides valuable insights, it can occasionally produce false signals, especially during volatile market conditions.
- Not Foolproof: Despite its predictive nature, no indicator, including the IMI, can guarantee future price movements. It’s essential to use the IMI in tandem with other analytical tools and maintain sound risk management practices.
Coding it in Popular Trading Languages
In the era of algorithmic trading, coding technical indicators has become an essential skill. The IMI, despite its complex nature, can be coded in popular trading languages like Python and R relatively easily. While the specifics of the code can vary, the core concept involves creating a function that calculates the gains and losses, sums them up over a specified period, and then calculates the IMI as a percentage.
Intraday Momentum Index (IMI) in R
This is how you might code it in R. This code assumes you have a data frame named data
with columns Open
and Close
representing the opening and closing prices for each day.
# Function to calculate gains and losses
calculate_gains_losses <- function(open, close) {
gains <- ifelse(close > open, close - open, 0)
losses <- ifelse(open > close, open - close, 0)
return(list(gains = gains, losses = losses))
}
# Function to calculate IMI
calculate_IMI <- function(data, n = 14) {
gains_losses <- calculate_gains_losses(data$Open, data$Close)
gains <- gains_losses$gains
losses <- gains_losses$losses
# Calculate sums of gains and losses over the period
sum_gains <- rollapply(gains, width = n, FUN = sum, align = "right", fill = NA)
sum_losses <- rollapply(losses, width = n, FUN = sum, align = "right", fill = NA)
# Calculate IMI
IMI <- (sum_gains / (sum_gains + sum_losses)) * 100
return(IMI)
}
# Add IMI to data
data$IMI <- calculate_IMI(data)
This code uses the rollapply
function from the zoo
package to calculate the rolling sums of gains and losses which needs to be loaded or installed. The calculate_IMI
function then calculates the IMI for each period.
Remember to handle NA values that will be generated for the first few rows (since the rolling sum cannot be calculated for these rows) in a way that makes sense for your analysis.
Intraday Momentum Index in Python
Let’s say we want to go further and create the index in Python for a particular stock, such as Palantir and then print it on an intraday candlestick chart using the free software VSCode. You can do this using Python, pandas, yfinance to download the stock data, and mplfinance to create the candlestick chart. This code assumes you have these libraries installed. If not, download VSCode follow the step by step instructions in it to install and use Python then you can install the libraries using pip by writing this in the Terminal window at the base of it:
pip install pandas yfinance mplfinance
The Python code would then be:
import pandas as pd
import yfinance as yf
import mplfinance as mpf
# Download historical data for desired ticker symbol (Palantir in this case)
ticker = "PLTR"
data = yf.download(ticker, period="5d", interval="15m")
# Calculate gains and losses
data['Gains'] = (data['Close'] - data['Open']).clip(lower=0)
data['Losses'] = (data['Open'] - data['Close']).clip(lower=0)
# Calculate IMI
data['IMI'] = (data['Gains'].rolling(window=14).sum() /
(data['Gains'].rolling(window=14).sum() + data['Losses'].rolling(window=14).sum())) * 100
# Add candlestick chart in the first row
ap = mpf.make_addplot(data['IMI'], panel=1, color='purple')
mpf.plot(data, type='candle', title='Palantir Stock Price', addplot=ap)
This script first downloads the past 5 days’ 15 minute periods data for Palantir. It then calculates the gains and losses for each period and uses these to calculate the IMI. Finally, it creates a 15 minute candlestick chart of the stock price and places the IMI on a panel beneath. You can execute it in VSCode by pressing the play icon in the top right of the software.
Note that NA values that will be generated for the first few rows (since the rolling sum cannot be calculated for these rows).
Python IMI Chart Output
The output should then look something like mine with the IMI percentage reading represented by a line in the lower panel:
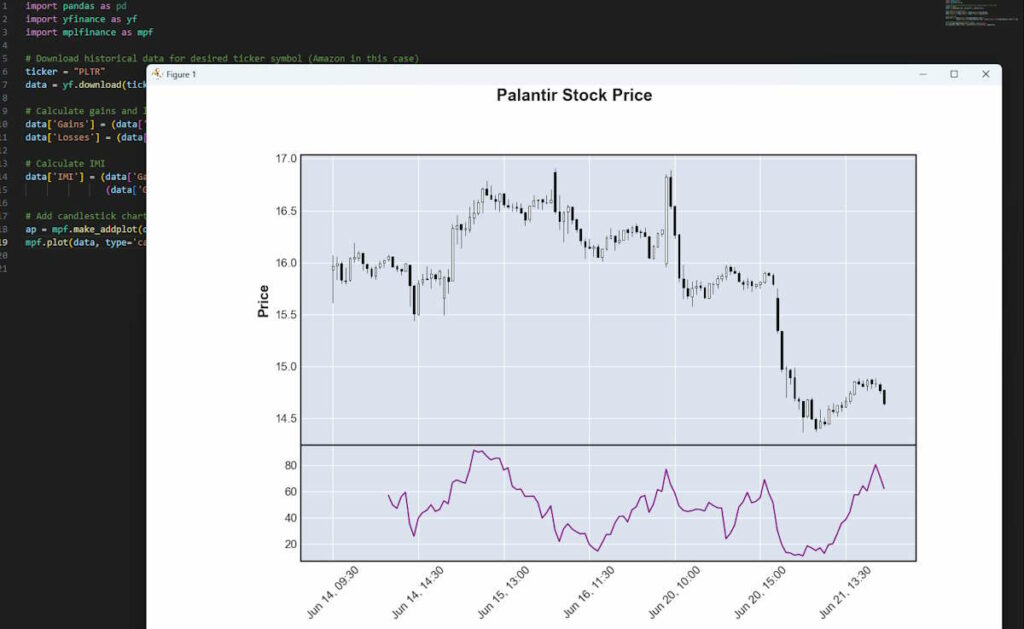
If you were looking to sell around readings over 80% and buy on readings under 20% at first glance you wouldn’t have done too badly.
Key Takeaways
- Intraday Momentum Index (IMI): A technical tool that blends candlestick analysis with the relative strength index (RSI) to offer overbought or oversold signals, aiding in informed trading decisions.
- Origins: Conceived by Tushar Chande in the 1990s to cater to the need for an intraday market momentum indicator.
- Mathematical Construction: The IMI is a percentage derived from comparing the gains and losses over a specific period, providing an indication of market momentum.
- Purpose: It helps identify potential overbought or oversold conditions in the market, assisting traders in pinpointing potential entry and exit points.
- Advantages and Limitations: The IMI provides crucial insights into intraday market momentum as a leading indicator. However, for optimal results, it should be paired with other indicators and analytical tools.
- Coding the IMI: It can be coded in popular trading languages like Python and R as we have demonstrated above, allowing for the creation of a candlestick chart of the stock price with the IMI displayed beneath it.
Further Reading
“Technical Analysis Explained: The Successful Investor’s Guide to Spotting Investment Trends and Turning Points“ by Martin J. Pring. This book is a comprehensive guide to technical analysis and covers a wide range of topics, including various technical indicators.
“Evidence-Based Technical Analysis: Applying the Scientific Method and Statistical Inference to Trading Signals“ by David Aronson. This book applies rigorous statistical techniques to evaluate the performance of various technical indicators and trading strategies.
“Quantitative Trading: How to Build Your Own Algorithmic Trading Business“ by Dr. Ernest Chan. This book provides insights into the world of algorithmic trading and covers various aspects of coding trading strategies.
“A Complete Guide to the Futures Market: Technical Analysis, Trading Systems, Fundamental Analysis, Options, Spreads, and Trading Principles“ by Jack D. Schwager and Mark Etzkorn. This book provides a comprehensive overview of futures trading, including technical analysis and trading systems.
“Advances in Financial Machine Learning“ by Marcos Lopez de Prado. This book covers various aspects of machine learning in finance, including the use of technical indicators in machine learning models.
“The Evaluation and Optimization of Trading Strategies“ by Robert Pardo. This book provides a step-by-step process for developing and validating trading strategies, including the use of technical indicators.
“Technical Analysis of the Financial Markets: A Comprehensive Guide to Trading Methods and Applications“ by John J. Murphy. This book is a classic in the field of technical analysis and covers a wide range of topics, including various technical indicators.
Leave a Reply